Before the Pi's UART can be used for this purpose, there are some preparations that need to be made. Please refer to http://wei48221.blogspot.tw/2016/08/how-to-use-uart-of-raspberry-pi-for_99.html for info. on how to prepare the Pi's UART.
Schematic:
Checking the version of the installed Python.
Issue "python --version".
Install pySerial
Issue "sudo apt-get install python-serial".
Other preparation works
Create a folder call "python" (if it hasn't been created) to keep the python programs.
1. Issue "mkdir python" to create the python folder.
2. Issue "ls" to make sure the folder is created.
Change working directory to the newly created python folder.
"cd python"
Launch nano text editor and name the file created "serial_read.py"
"nano serial_read.py"
Code
Type in the code between the 2 dash lines.
----------------------------------------------------------------------------------------------------------------------
#!/usr/bin/env pythonimport time
import serial
ser = serial.Serial(
port='/dev/ttyAMA0',
baudrate = 9600,
parity=serial.PARITY_NONE,
stopbits=serial.STOPBITS_ONE,
bytesize=serial.EIGHTBITS,
timeout=1
)
counter=0
while 1:
x=ser.readline()
print x
---------------------------------------------------------------------------------------------------------------------
IMPORTANT!!
Watch out for the indent as python uses spacing at the start of the line to determine when code blocks start and end (http://stackoverflow.com/questions/1016814/what-to-do-with-unexpected-indent-in-python).
Save the program.
Ctrl-X to exit the editing mode, then "Y" to save the file.
Running the program
"python serial_read.py".
Here I have an Arduino Uno with some sensors and a HC-11 module attached to it. Whenever a sensor is triggered, the Uno will send a message out via the HC-11 module. The message is picked up by the "serial_read.py " running on the Raspberry Pi and displayed in the PuTTY terminal.
When we are done with the program, use Ctrl-C to stop its execution.
References:
Using UART on Raspberry Pi – Python
https://electrosome.com/uart-raspberry-pi-python/
Arduino Lesson 17. Email Sending Movement Detector
https://learn.adafruit.com/arduino-lesson-17-email-sending-movement-detector/python-code
Read and write from serial port with Raspberry Pi
http://www.instructables.com/id/Read-and-write-from-serial-port-with-Raspberry-Pi/
---------------------------------------------------------------------------------------------------------------------
Product Offered:
Note, the 2 modules listed here have been tested to work with Arduino Uno and Raspberry Pi.
433MHz Serial RF Module -- HC-11
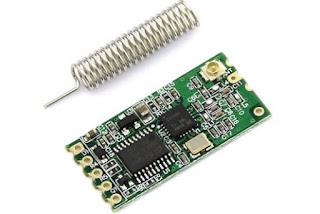
- Price: US$6.99/ea;
- Item Location: Taipei, Taiwan;
- Range: ~100 Meters (direct line of sight without obstruction);
- Frequency: 433MHz;
- Operating Voltage: DC 3.3V ~ 5V (when feeding DC 3.3V to the VCC of the module, the module works with Raspberry Pi without the need for level shifting / voltage division);
- Serial baud rate: 1.2Kbps to 115.2Kbps (default 9.6Kbps);
- Interface protocol: UART/TTL;
- At least 2 HC-11 modules are needed to form a complete wireless link.
IMPORTANT - THIS MODULE DOESN'T WORK WITH THE 433MHz Serial RF Module HC-12.
-------------------------------------------------------------------------------------------------------------------------
433MHz Serial RF Module -- HC-12
- Price: US$7.99/ea;
- Item Location: Taipei, Taiwan;
- Range: ~1000 Meters (@ 5000bps) direct line of sight without obstruction);
- Frequency: 433MHz;
- Operating Voltage: DC 3.3V ~ 5V (when feeding DC 3.3V to the VCC of the module, the module works with Raspberry Pi without the need for level shifting / voltage division);
- Serial baud rate: 1.2Kbps to 115.2Kbps (default 9.6Kbps);
- Interface protocol: UART/TTL;
- At least 2 HC-12 modules are needed to form a complete wireless link.
IMPORTANT - THIS MODULE DOESN'T WORK WITH THE 433MHz Serial RF Module HC-11.
How to purchase:
Leave your message in the comment section below or send me an e-mail. I will contact you for detail.
Nice tutorial,,
ReplyDeleteHow to set HC(11/12) module freq for both Arduino & Raspi to be able communicate ?
Thx
By default, the modules are set to the same frequency so they could talk to each other right out of the box. However, it's possible to change the frequency when it's needed..
Delete